Node Rush: The Intersection of Strategic Fun and Technical Innovation
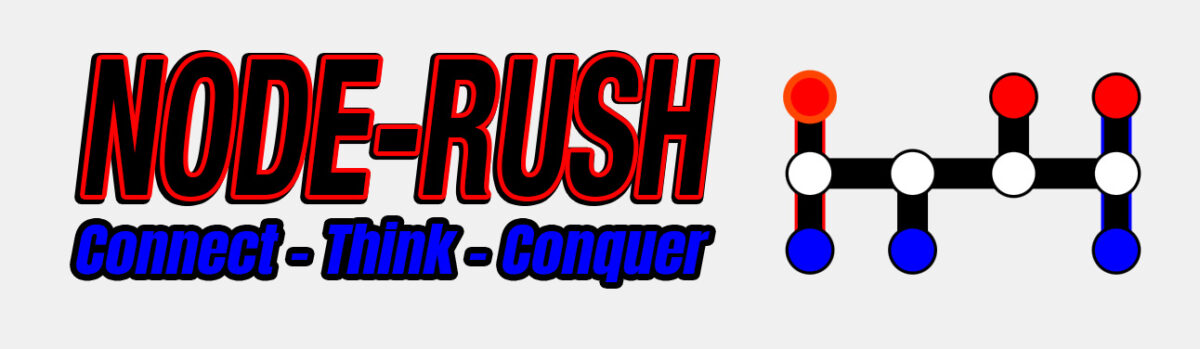
Node Rush isn’t just a game—it’s a well-crafted experience designed to combine the timeless thrill of strategic board games with modern technology. It’s a project born from the love of classic games, enriched with artificial intelligence and made accessible to everyone through a web browser. This portfolio entry will take you through the evolution of Node Rush, from concept to completion, shedding light on its design, development, and the technical feats that make it unique.
NODE RUSH
The Concept: Breathing New Life into Classic Strategy
The inspiration behind Node Rush was to capture the essence of classic board games—the simplicity, the strategic depth, and the ability to challenge oneself or an opponent. At its core, Node Rush is about two players strategizing to reach their respective goals on a graph-like board, using node connections to advance their positions. One can see it as a homage to traditional board games, but it’s powered by the web, AI, and scalable graphics.
Node Rush’s gameplay is designed to be intuitive: each player has a set of nodes they must move across the board. Players alternate turns, selecting nodes and advancing towards their victory conditions while blocking their opponent’s moves. This deceptively simple concept offers surprising depth, making Node Rush a game that’s easy to learn but difficult to master.
Crafting the Game Board and User Interface
Building a visually appealing and highly responsive game board was one of the key goals of Node Rush. The decision to use Scalable Vector Graphics (SVG) for the board was intentional. SVG allowed the game visuals to be clean and crisp on any screen size, ensuring consistency and sharpness whether the game was being played on a phone, tablet, or desktop. SVG also provided direct access to manipulate individual nodes and paths with JavaScript, enabling complex interactions while keeping performance smooth.
The board design wasn’t just about aesthetics. Each node and connection on the board was made interactive, allowing players to simply click (or tap) to make a move. This was made possible through JavaScript, which handled event listeners for node selection and path highlighting. For instance, when a player selects a node, the surrounding valid moves are visually highlighted to guide them. This interactivity makes the game accessible even to those who might be unfamiliar with strategic board games, reducing the learning curve.
// Example of adding click event listeners to SVG nodes
nodes.forEach((node) => {
node.addEventListener("click", (e) => {
e.stopPropagation(); // Prevent unintended board clicks
if (gameOver) return; // Stop interaction if the game is over
if (currentPlayer === PLAYERS.PLAYER2) return; // Prevent actions during AI's turn
if (selectedToken) {
if (selectedToken === node) {
clearSelection();
return;
}
moveToken(selectedToken, node);
clearSelection();
} else if (isCurrentPlayerToken(node)) {
clearSelection();
selectedToken = node;
node.classList.add("selected");
highlightValidMoves(node);
}
});
});
The user interface was carefully designed to ensure a responsive experience across different devices. CSS media queries were utilized to adjust the scaling, ensuring a consistent look and feel regardless of the screen dimensions. Controls like game reset buttons, rule pop-ups, and move indicators were designed to be easily accessible on any device, making Node Rush a true cross-platform experience.
Developing a Competitive AI: Intelligence That Learns
One of the highlights of Node Rush is its AI opponent, which provides a challenging yet fair adversary. The AI was designed using a combination of Minimax with Alpha-Beta pruning, a popular technique used in two-player games. The goal was to make the AI competitive without feeling impossible to beat. This required finding a balance between calculating deep into the game tree and making quick decisions to ensure a smooth, uninterrupted player experience.
The AI employs a heuristic evaluation function that considers multiple factors, such as node control, blocking opponent moves, and advancing towards its goal. To achieve different difficulty levels, the AI uses iterative deepening, adjusting its depth based on available computation time.
/**
* Gets the best move for the AI within a time limit.
* @param {Object} gameState - The current game state
* @param {number} timeLimit - Time limit in milliseconds
* @returns {Object|null} - The best move or null if no move is available
*/
getBestMove(gameState, timeLimit = 1000) {
const startTime = performance.now();
let bestMove = null;
let depth = 1;
// Iterative deepening
while (depth <= this.maxDepth) {
const timeElapsed = performance.now() - startTime;
if (timeElapsed >= timeLimit) {
break;
}
const result = this.minimax(gameState, depth, -Infinity, Infinity, true, startTime, timeLimit);
if (result && result.move) {
bestMove = result.move;
}
depth++;
}
return bestMove;
}
One challenge was ensuring that the AI didn’t slow down gameplay on lower-end devices. To solve this, the AI’s decision-making depth is adjusted dynamically based on the time taken, and some less critical calculations are skipped in favor of maintaining the flow of the game.
Ensuring a Great User Experience: Design and Details
The user experience of Node Rush was prioritized from the start. Beyond simply playing a game, the goal was to create an engaging experience that felt polished and responsive. To do this, I implemented features like move highlighting, where valid moves are illuminated, and selection indicators, which help guide the player through each turn.
The UI displays real-time game statistics, including move count and time elapsed, giving players a sense of accomplishment and a competitive edge. The timer creates a sense of urgency, while the move counter encourages players to strategize efficiently.
<div class="stats-container">
<div id="move-counter" style="display: flex; align-items: center; gap: 8px">
<svg width="16" height="16" viewBox="0 0 24 24">
<circle cx="12" cy="12" r="10" fill="#ff0000" />
</svg>
<span id="move-count-text" style="font-weight: bold">Moves: 0</span>
</div>
<div id="timer" style="display: flex; align-items: center; gap: 8px">
<svg width="16" height="16" viewBox="0 0 24 24" fill="none" stroke="currentColor" stroke-width="2">
<circle cx="12" cy="12" r="10" />
<polyline points="12 6 12 12 16 14" />
</svg>
<span id="timer-text" style="font-weight: bold">Time: 0:00</span>
</div>
</div>
Tackling Technical Challenges: Performance Optimization
Creating a browser-based game presents unique challenges, particularly in terms of performance and compatibility. Since Node Rush runs entirely within the browser, it was critical to ensure that all interactions were fluid, regardless of the device. To achieve this, I optimized the rendering pipeline by leveraging requestAnimationFrame() to synchronize DOM updates with the browser’s refresh cycle, reducing redundant redraws and ensuring smooth transitions.
function moveToken(fromNode, toNode) {
if (isAdjacent(fromNode, toNode) && !isOccupied(toNode)) {
if (!timerStarted) {
startTimer();
}
fromNode.setAttribute("fill", "white");
toNode.setAttribute("fill", currentPlayer === PLAYERS.PLAYER1 ? "red" : "blue");
moveCount++;
updateMoveCounter();
if (!checkWinCondition()) {
currentPlayer = currentPlayer === PLAYERS.PLAYER1 ? PLAYERS.PLAYER2 : PLAYERS.PLAYER1;
updatePlayerStatus();
checkFrozenAndMove();
}
}
}
Moreover, the AI computations were optimized to avoid blocking the main thread, keeping the user interface responsive. Caching the adjacency list and pre-calculating paths allowed for faster access to game data during AI evaluations.
Beyond Gameplay: Expanding Node Rush
The success of a game often lies in its ability to evolve and stay engaging over time. Node Rush was designed with the future in mind. Potential additions include a multiplayer mode, where players can compete against each other, adding a social aspect to the game. Additionally, new game modes could introduce obstacles, power-ups, or different board configurations to keep players on their toes.
Another idea is to implement a campaign mode, where players must complete a series of increasingly challenging boards. Each level could add new mechanics—like restricted paths, dynamic obstacles, or even power-ups that can be used strategically to influence the outcome.
The groundwork has also been laid for adding leaderboards. Players could soon compete for the best times or fewest moves, providing an additional layer of motivation to master the game.
Conclusion: A Synthesis of Strategy and Technology
Node Rush is more than just a game; it is a modern reinvention of classic board game mechanics, designed to be accessible yet challenging. It seamlessly blends artificial intelligence, scalable graphics, and responsive web technologies into an experience that captivates players of all skill levels. The game’s evolution has been driven by a passion for strategy and technology—turning a simple idea into something enjoyable and enduring.
From the interactive SVG-based game board to the intelligent AI that adapts and challenges players, every aspect of Node Rush has been crafted with attention to detail and care. The journey of creating Node Rush was not just about building a game but about pushing the boundaries of what a browser-based game could achieve.
Whether you’re looking to relax with a casual game or challenge yourself against an intelligent opponent, Node Rush offers something for everyone. Dive in, make your moves, and experience the joy of strategy—right from your browser.
Stay tuned as Node Rush continues to grow, with more features and modes on the horizon, ensuring there’s always something new to discover.